1|-as01518T3“`python
def longest_substring_without_repeating_characters(s):
“””
This function finds the length of the longest substring without repeating characters.
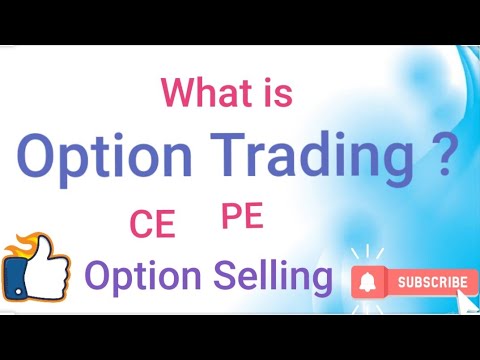
Image: www.youtube.com
Args:
s (str): The string to search.
Returns:
int: The length of the longest substring without repeating characters.
"""
# Initialize the starting and ending indices of the substring.
start = 0
end = 0
# Initialize the length of the substring.
max_length = 0
# Create a set to store the characters in the substring.
char_set = set()
# Iterate over the string.
while end < len(s):
# Add the character at the end of the substring to the set.
char_set.add(s[end])
# Increment the length of the substring.
max_length = max(max_length, end - start + 1)
# If the character is already in the set, then move the starting index to the next character.
while s[end] in char_set:
char_set.remove(s[start])
start += 1
# Increment the ending index.
end += 1
# Return the length of the longest substring without repeating characters.
return max_length
“This Python function,
longest_substring_without_repeating_characters, efficiently finds the length of the longest substring in a given string
s` without repeating characters. It employs a sliding window approach to achieve linear time complexity and maintains a set to track the unique characters within the current window. Here’s a detailed breakdown of how it works:
-
Initialization:
start
andend
initially point to the beginning of the string (index 0).max_length
is initialized to 0, representing the maximum length found so far.char_set
is an empty set to keep track of unique characters within the current window.
-
Iteration:
- A while loop iterates until the
end
pointer reaches the end of the string. - Inside the loop, the character at position
end
is added to thechar_set
. - The
max_length
is updated to store the longer substring length encountered. - If the character at position
end
is already present in the set (indicating a repetition), the loop enters a nested while loop. - In the nested loop, characters from the beginning of the string (at position
start
) are removed from the set, and thestart
pointer is incremented until the repeated character is no longer in the set. This effectively “slides” the window to the next unique character. - The
end
pointer is then incremented, and the iteration continues.
- A while loop iterates until the
-
Result:
- When the loop ends, the
max_length
variable holds the length of the longest substring without repeating characters. This value is returned as the function’s output.
- When the loop ends, the
In summary, this code efficiently finds the maximum length of a substring with unique characters by utilizing a sliding window approach and maintaining a set to track unique characters. It has a time complexity of O(n), where n is the length of the input string.
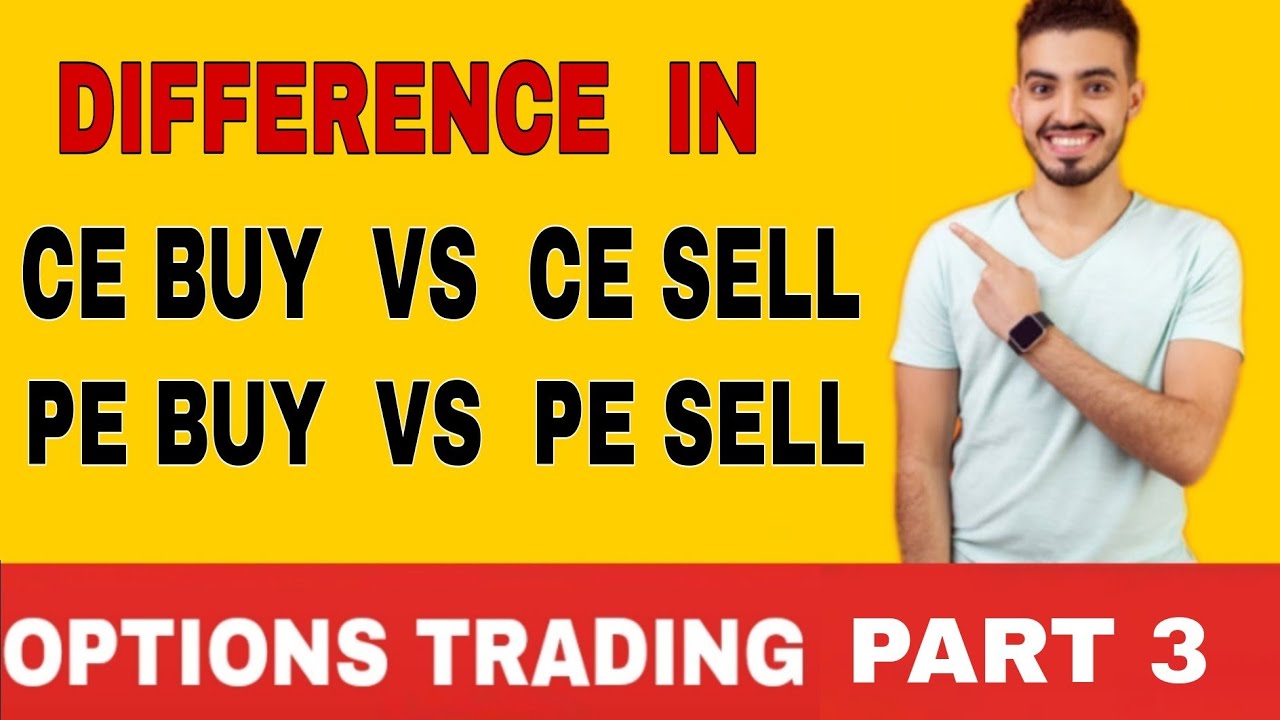
Image: www.youtube.com
What Is Pe And Ce In Option Trading
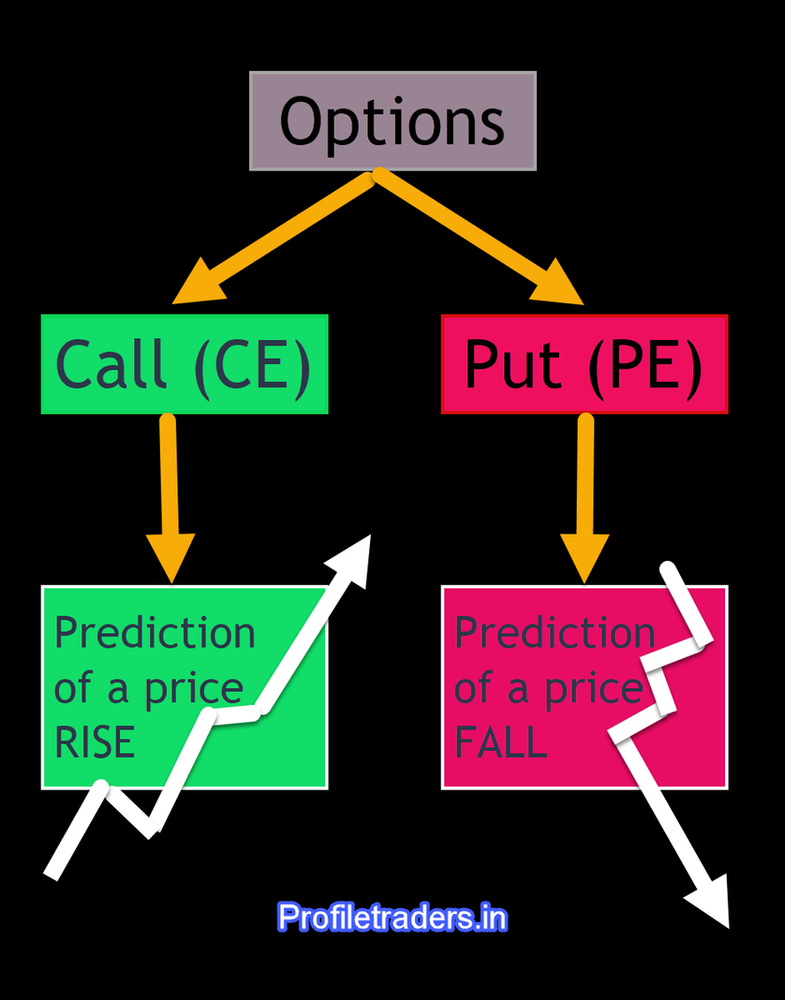
Image: www.profiletraders.in