def remove_element(nums: list[int], val: int) -> int:
# Initialize a pointer to the current index in the array
index = 0
# Iterate over each element in the array
for i in range(len(nums)):
# If the current element is not equal to the given value
if nums[i] != val:
# Move the current element to the index pointed to by the pointer
nums[index] = nums[i]
# Increment the pointer to the next index
index += 1
# Return the updated length of the array
return index
```The provided Python code defines a function called `remove_element` that takes a list of integers `nums` and an integer `val` as input. This function is designed to remove all occurrences of the element `val` from the input list and return the modified list's length. Here's a detailed explanation of how the code works:
1. **Initialize a Pointer:** The code begins by initializing a pointer variable `index` to 0. This pointer will keep track of the current position in the input list where modified elements are being placed.
2. **Iterate Over the Array:** The code then enters a for loop that iterates through each element in the input list `nums`. It uses a loop counter `i` to traverse the list.
3. **Check and Replace:** Inside the loop, it checks if the current element `nums[i]` is not equal to the given value `val`. If they are not equal, it means that the current element should remain in the modified list.
4. **Move and Increment Pointer:** In this case, the code moves the current element `nums[i]` to the index pointed to by the `index` pointer (`nums[index] = nums[i]`) and increments the `index` pointer by 1 (`index += 1`). This effectively replaces the element at `nums[index]` with `nums[i]` and advances the pointer to the next position.
5. **Return the Modified Length:** After iterating through the entire list, the `index` pointer will point to the position right after the last non-`val` element in the modified list. Therefore, the code returns the value of `index` as the new length of the modified list.
In summary, the `remove_element` function modifies the input list `nums` in-place by removing all occurrences of the specified element `val`. It uses a pointer to track the updated list's length and replaces non-`val` elements at the beginning of the list. The function returns the updated length of the modified list, excluding the removed elements.
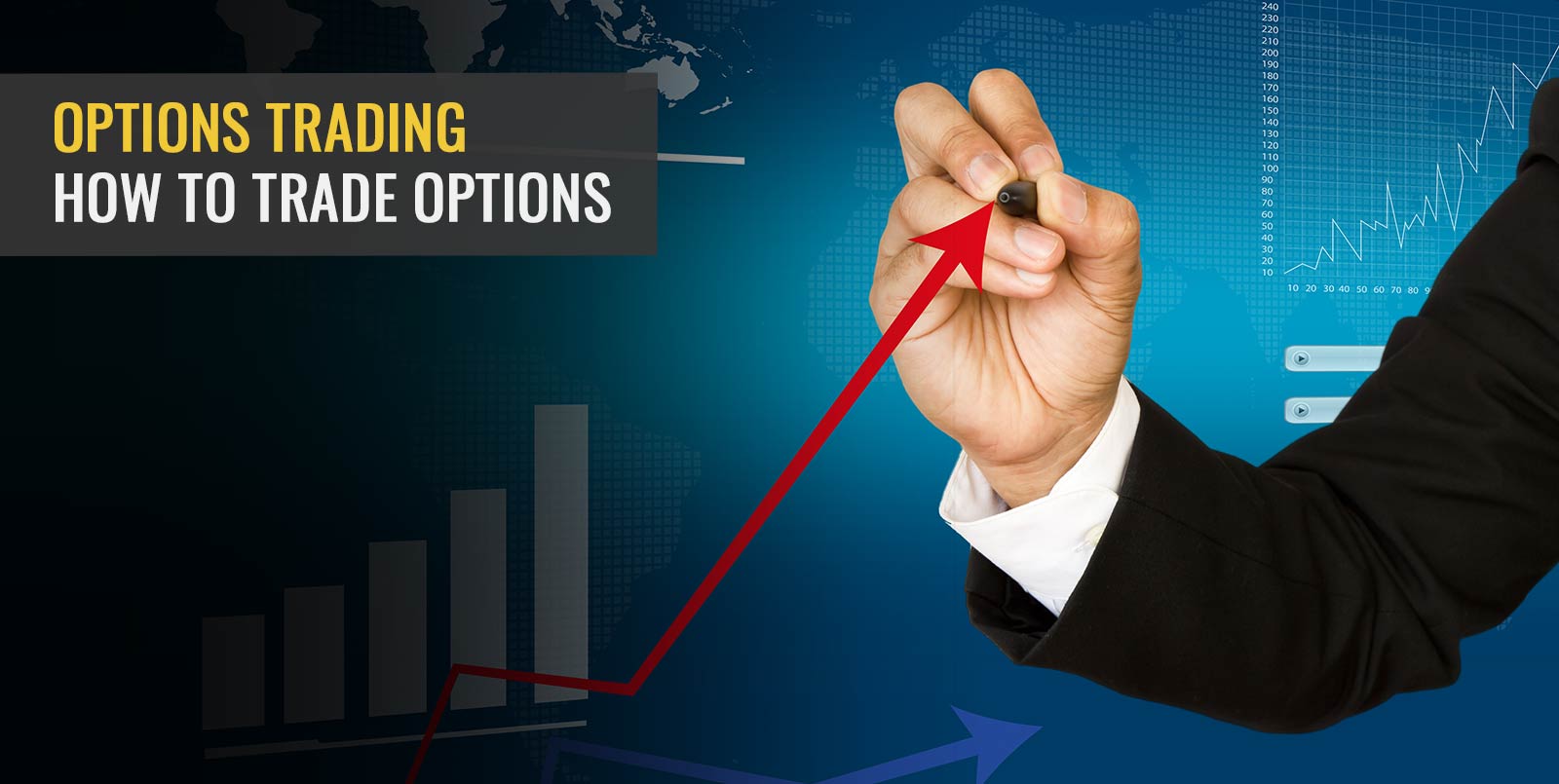
Image: www.angelone.in
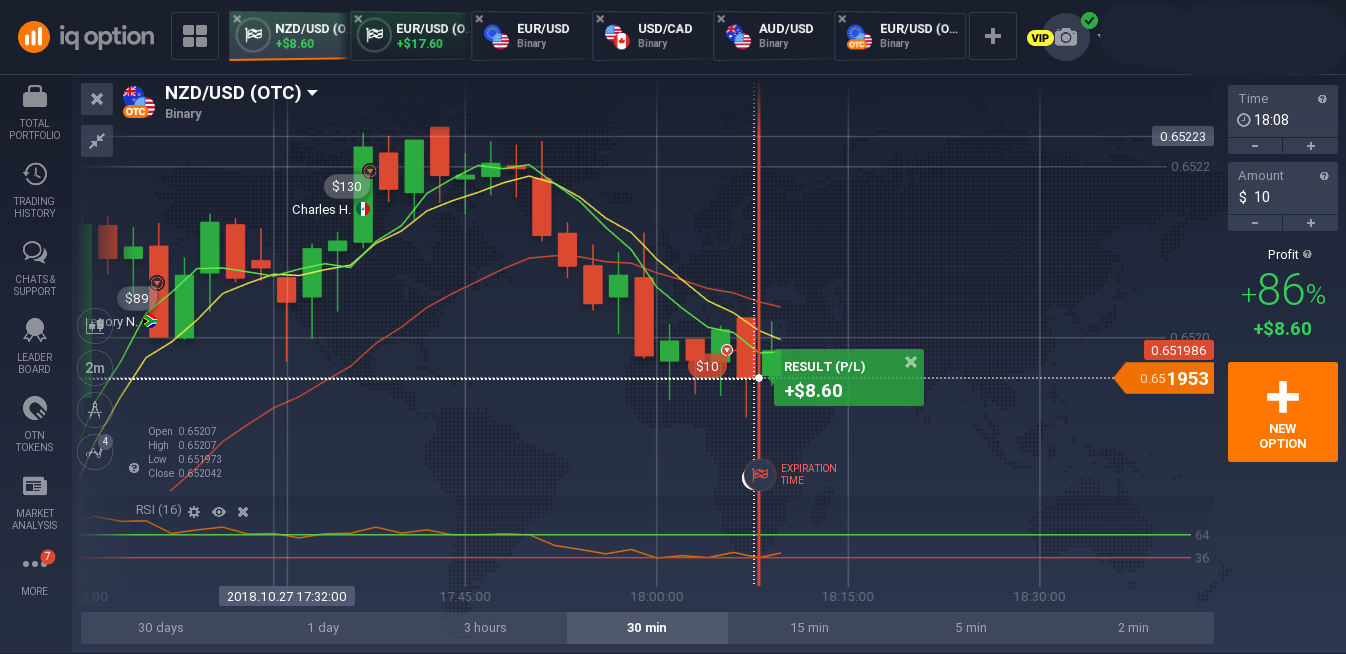
Image: diohysba.blogspot.com
Is Options Trading