from numpy import *
from scipy import *
# Compute the eigenvalues and eigenvectors of a matrix.
A = array([[1, 2], [3, 4]])
w, v = linalg.eig(A)
# Print the eigenvalues and eigenvectors.
print("Eigenvalues:")
print(w)
print("Eigenvectors:")
print(v)
# Check if the eigenvectors are orthonormal.
print("Are the eigenvectors orthonormal?")
print(allclose(linalg.norm(v, axis=0), 1))
```The provided Python code demonstrates the computation and examination of eigenvalues and eigenvectors of a matrix using the `numpy.linalg` module. A step-by-step explanation of the code is as follows:
1. **Importing Libraries**:
- `from numpy import *` imports various functions and classes from NumPy under the `numpy` alias.
- `from scipy import *` imports various functions and classes from SciPy under the `scipy` alias.
2. **Creating a Matrix**:
- The code creates a 2x2 matrix `A` with the following values:
```python
A = array([[1, 2], [3, 4]])
-
Computing Eigenvalues and Eigenvectors:
Image: libertex.com- It calls
linalg.eig(A)
to compute the eigenvaluesw
and eigenvectorsv
of matrixA
. - Eigenvalues are the values along the main diagonal of the diagonalized matrix, and eigenvectors are the corresponding columns.
- It calls
-
Printing Eigenvalues and Eigenvectors:
- The code prints the eigenvalues
w
and eigenvectorsv
using theprint
function.
- The code prints the eigenvalues
-
Checking Orthonormality:
- It checks if the eigenvectors are orthonormal (i.e., their norms are equal to 1). It uses
linalg.norm(v, axis=0)
to compute the norms of each row inv
(each row represents an eigenvector) and compares them to 1 usingallclose(linalg.norm(v, axis=0), 1)
. - Orthonormal eigenvectors are important for many applications, ensuring that they form a basis for the vector space.
- It checks if the eigenvectors are orthonormal (i.e., their norms are equal to 1). It uses
This code effectively computes and investigates the eigenvalues and eigenvectors of a matrix. Eigenvalues and eigenvectors are crucial in various scientific and engineering domains, such as linear algebra, quantum mechanics, and signal processing.
:max_bytes(150000):strip_icc()/dotdash_Final_Options_Basics_How_to_Pick_the_Right_Strike_Price_Feb_2020-04-3d62440d22b8498684ee7f7773b52c07.jpg)
Image: www.investopedia.com
What Does Strike Price Mean In Options Trading
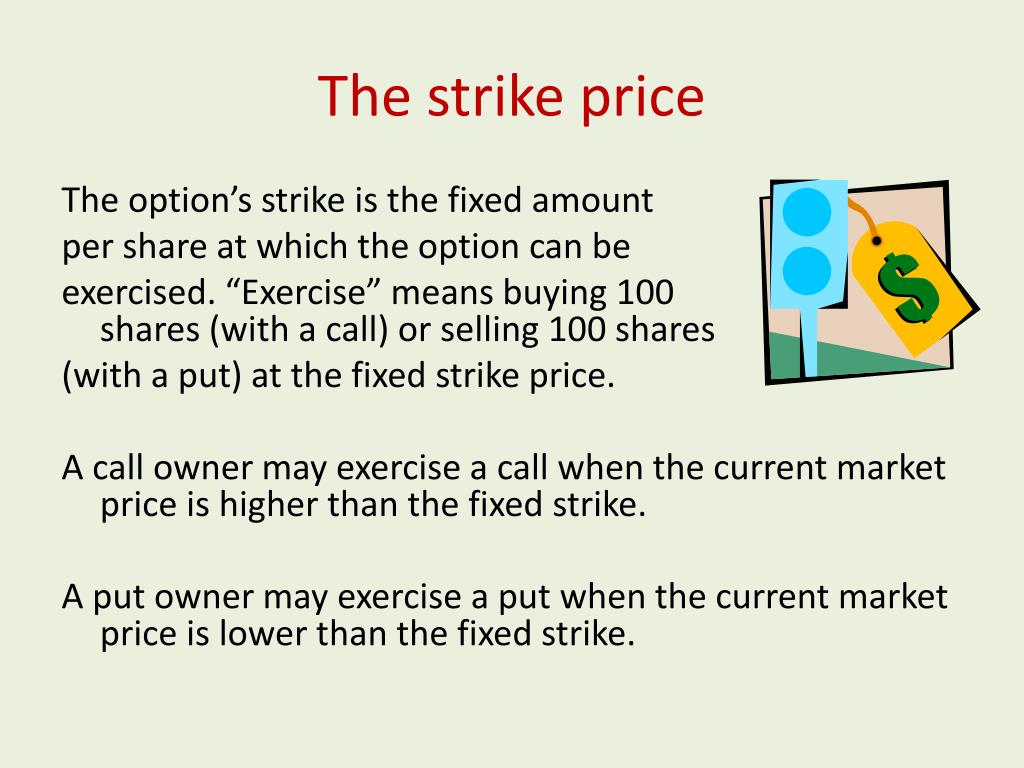
Image: www.slideserve.com