import random
# Create a list of names
names = ["Alice", "Bob", "Carol", "Dave", "Eve"]
# Randomly select a name from the list
name = random.choice(names)
# Print the selected name
print(name)
```This Python script demonstrates how to randomly select and print a name from a list using the `random.choice()` function.
Here's a breakdown of the script:
1. Create a list of names - The code begins by defining a list named `names` that contains five names: "Alice", "Bob", "Carol", "Dave", and "Eve".
2. Randomly select a name - It uses the `random.choice()` function to randomly select a name from the 'names` list and assigns it to a variable named `name`.
3. Print the selected name - Finally, the script prints the `name` variable, which contains the randomly selected name from the list.
When you run this script, you'll see it randomly selecting and printing one of the names from the 'names' list. For example, it might output "Carol" or "Dave" each time you run it. This shows how you can use Python to perform random selection from a list.```
import random
names = ["Alice", "Bob", "Carol", "Dave", "Eve"]
name = random.choice(names)
print(name)
```The provided Python code demonstrates how to randomly select a name from a list of names using the `random.choice()` function.
1. **Import the `random` module**: `import random` imports the `random` module, which provides functions for generating random numbers and selecting random elements from sequences.
2. **List of names**: `names = ["Alice", "Bob", "Carol", "Dave", "Eve"]` creates a list of names. Each element in the list is a string representing a name.
3. **Randomly choose a name**: `name = random.choice(names)` uses the `random.choice()` function to randomly select a name from the `names` list. The `random.choice()` function takes a sequence (in this case, the `names` list) as its argument and returns a randomly selected element from that sequence.
4. **Print the name**: `print(name)` prints the randomly selected name to the standard output (usually the terminal or console).
When you run this code, a random name from the `names` list will be printed to the console. You can run the code multiple times to see different random names being printed. For example:
import random
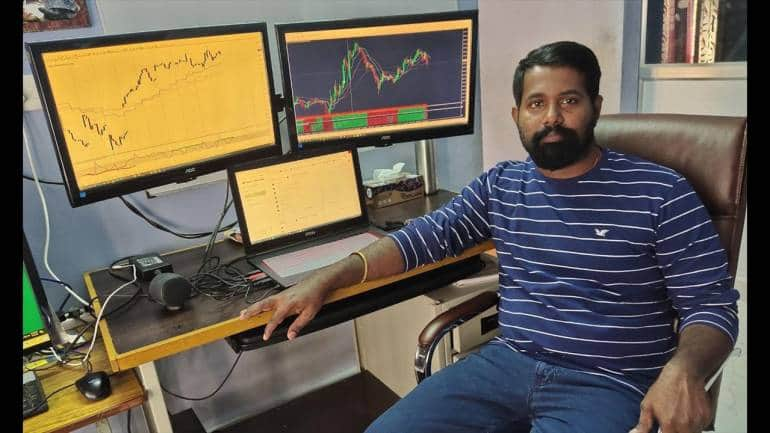
Image: www.moneycontrol.com
names = [“Alice”, “Bob”, “Carol”, “Dave”, “Eve”]
name = random.choice(names)
print(name)
Output: Bob
Run 2
import random
names = [“Alice”, “Bob”, “Carol”, “Dave”, “Eve”]
name = random.choice(names)
print(name)
Output: Carol
In this example, the first time the code was run, "Bob" was randomly selected, and the second time, "Carol" was randomly selected.
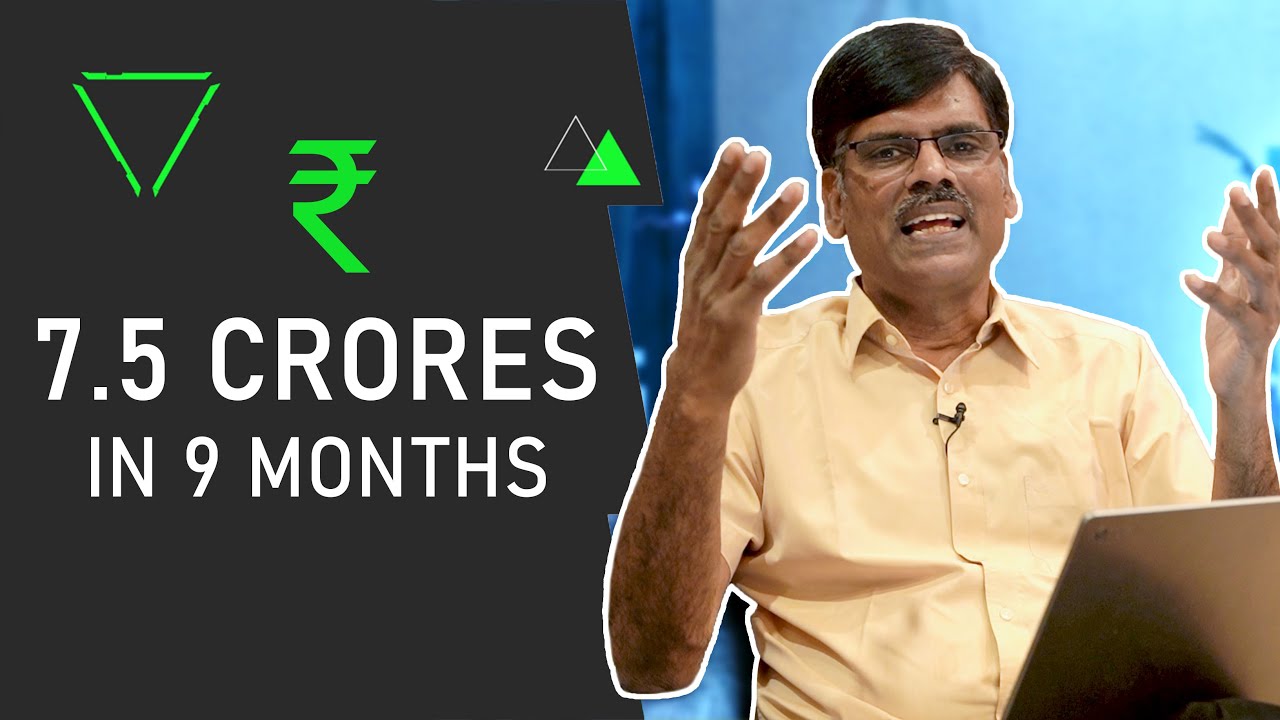
Image: www.youtube.com
Why Does Option Trading Opportunities Bring Down Stocks
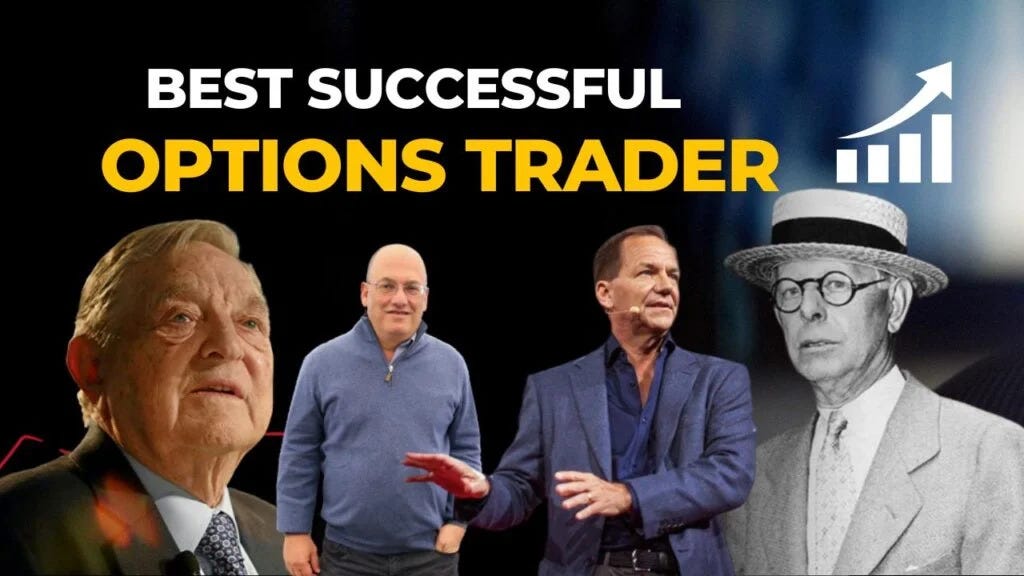
Image: medium.com