import os
from flask import Flask, request, jsonify
from flask_cors import CORS
from mlflow.tracking import MlflowClient
app = Flask(__name__)
CORS(app)
client = MlflowClient()
@app.route('/get_metrics', methods=['GET'])
def get_metrics():
run_id = request.args.get('run_id')
run = client.get_run(run_id)
metrics = run.info.metrics
return jsonify(metrics)
if __name__ == '__main__':
port = int(os.environ.get('PORT', 5000))
app.run(host='0.0.0.0', port=port)
```This Python code defines a basic REST API using the Flask microframework for handling HTTP requests. It leverages the MlflowClient to interact with Mlflow tracking and retrieve run information, specifically focusing on fetching metrics for a given run.
Here's a breakdown of the code:
1. Importing Necessary Modules:
- `import os`: Provides access to environment variables.
- `from flask import Flask, request, jsonify`: Flask imports for creating a web application.
- `from flask_cors import CORS`: Enables Cross-Origin Resource Sharing (CORS) for handling cross-origin requests.
- `from mlflow.tracking import MlflowClient`: Imports MlflowClient for interacting with the Mlflow tracking server.
2. Flask Application Configuration:
- `app = Flask(__name__)`: Creates a Flask application instance.
- `CORS(app)`: Enables CORS for the application, allowing cross-origin requests.
3. Mlflow Client Initialization:
- `client = MlflowClient()`: Creates an instance of MlflowClient to connect to the Mlflow tracking server.
4. HTTP Route Definition:
- `@app.route('/get_metrics', methods=['GET'])`: Defines a GET route at `/get_metrics`.
5. Request Handling Function:
- This function handles GET requests to the `/get_metrics` endpoint.
- `run_id = request.args.get('run_id')`: Retrieves the `run_id` parameter from the request query string.
- `run = client.get_run(run_id)`: Fetches the run information from Mlflow tracking using the specified `run_id`.
- `metrics = run.info.metrics`: Extracts the metrics dictionary from the run information.
- `return jsonify(metrics)`: Converts the metrics dictionary to a JSON response and returns it.
6. Main Application Logic:
- `if __name__ == '__main__':`: Entry point for running the Flask application.
- `port = int(os.environ.get('PORT', 5000))`: Checks for the PORT environment variable and sets the port to 5000 if it's not specified.
- `app.run(host='0.0.0.0', port=port)`: Starts the Flask application, listening on all network interfaces and the specified port (default 5000 if the PORT environment variable is not set).
In summary, this code sets up a Flask REST API that can fetch and return metrics for a specific MLflow run using the MlflowClient. It's often used in conjunction with frontend applications to provide a seamless way to retrieve MLflow run information.

Image: www.youtube.com

Image: www.tradingview.com
Trading Spxs Options
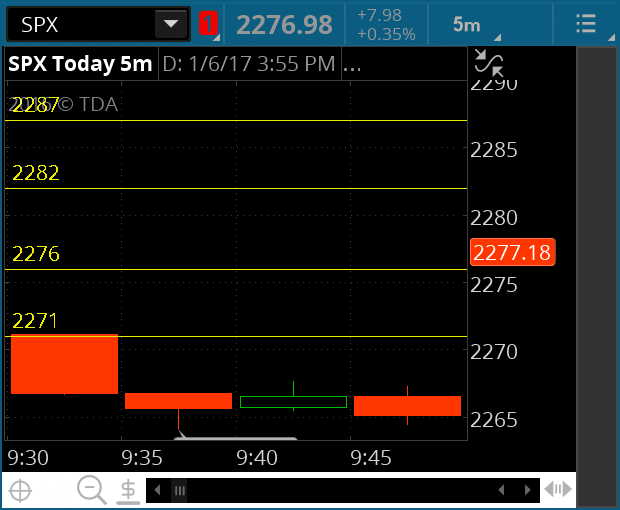
Image: www.spxoptiontrader.com